Basic Concepts of All Programming Computer Languages
Regardless of whether a computer program is written in “C”, Java, FORTRAN or AppleScript it will depend on four core concepts. They are:
- Variables
- Constants
- Types (of data)
- Flow Control – which includes:
- Repetitive Loops
- Conditional Branches
What is a variable?
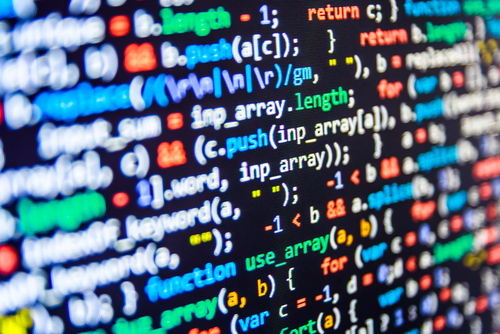
A variable is something that can vary while a program is running. It may be a checkbook balance that gets increased or decreased or the statistics of an athlete. Variables have names assigned by the programmer. Some languages have strict rules about the form of variable names, for instance they must begin with a “$”. In most languages variables can either be used everywhere in the program (global) or just in one part of the code (local.) Since global variables can be used throughout the program their names have to be unique. Local variables only exist within a specific section of the program and therefore can have the same name as other local variables in different program sections. The value of a variable is set while the program is running.
TIP: Many programmers start each global variable’s name with a “g” to indicate it’s a global variable.
What is a constant?
Constants are really just a way of assigning a name of a value. For instance, instead of having to enter 3.14159265358979323846 to use PI in calculations, our program can create a constant called
kPIand assign it the appropriate value. Saves a lot of typing and potential mistakes. The value of a constant is set when the program is “stored”.
TIP: Many programmers start each constant’s name with a “k” to indicate it’s a constant.
Types (of data)
The basic types of data are:
- Numbers (e.g. Integers & Real Numbers)
- Strings (e.g. “cat” & “800-111-1234”)
- Boolean (e.g. TRUE & FALSE)
Most programming languages handle and store different types of data differently. These languages use “strict typing” of variables and generally don’t allow code that does operations (e.g. addition) on different types. For instance: “c” + 7 would not be allowed because it doesn’t make strict sense to add a number to a word.
Other languages are more relaxed and change the types of variables in a statement to suit the need, if possible. They don’t all do this the same, though, so it’s important to keep in mind the type of data all your variables represent and the automatic conversions that the language performs. For instance
“c”+7 might result in
“c7” in one language and
“j” in another and
106 in yet another.
It’s a good idea to have a standard naming scheme to document what kind of variable each one is. For instance an identifying letter can be added to the end of the name of each variable to indicate its type.
Flow Control
Very few tasks can be achieved without decision points. These decision points might test a variable (such as creditcard_balance) and cause different parts of the program to run depending on the result. For instance:
IF creditcard_balance is greater than a certain amount
THEN send a notice to the card holder
ELSE don’t do anything.
There are several types of flow controls in most languages. They are:
- If/Then/Else (branching)
- Case statements (branching)
- For/Next (loops)
- Repeat While (loops)
- Repeat Until (loops)
Here is a very brief description of each:
Branching
- If/Then/Else loops do just what they sound like. It a variable meets a test condition, then something is done, otherwise something else is done.
- Case statements are like multiple IFs. The resulting action is based on which of several conditions (not just 2) the variable meets.
Loops
- For/Next loops repeat an action while automatically changing (generally increasing) the value of a counter variable.
- Repeat While loops repeat an action as long as some condition is TRUE.
- Repeat Until loops repeat an action until some condition is met.
Which kind of branching or looping you use is largely a matter of your programming style, although you’ll find in most cases, one type will make more sense than another.
These are the basic concept your need to understand any computer programming language. The specifics can be found in language references.